Elemental Agents with YAML files
Configuration of agents and agent teams in Elemental may be done in two different ways. First, Elemental is a python library and programmatic creation of agents and teams is simplified using AgentFactory
class. With specification of parameters one can quickly create agents and use them programmatically. Most complex workflows are meant to be handled in Elemental with orchestration of agent teams working in specific steps. This model is very generic and supports creation of the orchestrated team either programmatically or using YAML configuration file.
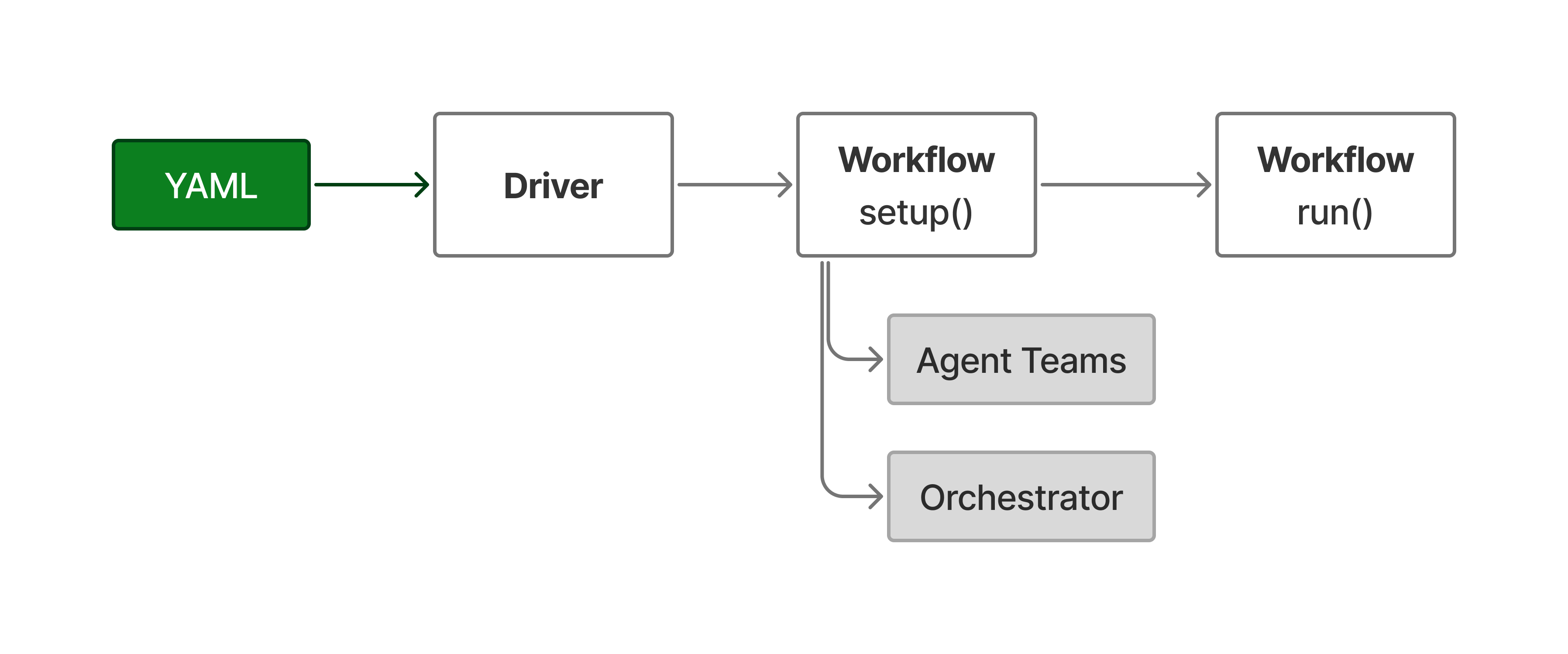
Main classes of Elemental that are involved in this process are Driver
and Workflow
. Driver
may be used to process and run YAML file and build in the created workflow into larger application. It is also main component behind the command line interface to Elemental. In the next sections we will use the command line interface to run the examples.
In this post we will show how to create agents and agent teams using YAML configuration files. We will show the configuration of a simple assistant, ReAct agent and a planner agent. The last one is a bit more complex and requires some explanation.
Simple Assistant
In the previous post we have introduced an example where the configuration of an agent (or an assistant, since we did not use any tools) was done using a YAML file. Our example was:
workflowName: ModelTest
workflow:
- executor
executor:
- name: Assistant
type: Simple
persona: >-
You are expert researcher and great
communicator of complex topics using
simple terms. You always give comprehensive
and extensive responses.
tools: []
llm: ollama|qwen3:4b
temperature: 0
frequencyPenalty: 0
presencePenalty: 0
topP: 1
maxTokens: 2000
stopWords: <PAUSE>, STOP
with this difference, that above we have skipped the template
section. If this section is not present, Elemental will use the default prompt template from file. For any type of agent, you can overwrite the default prompt including the template
section in the configuration.
All example configurations given here are also available in the Elemental GitHub repository in the examples folder.
This simple assistant can be run using the Elemental command line interface with:
python -m elemental_agents.main.elemental --config examples/06-yaml-config-file/config-example.yaml
Agents using Tools
In this example we will take the same assistant and add a few tools to it. To enable our agent to use tools, we will also change the type of the agent from type: Simple
to type: ReAct
. The ReAct agent is a reasoning agent that uses the tools to perform actions. The tools are used in the <action>
section of the response. The agent will also give its reasoning in the <thought>
section.
workflowName: ReAct Agent
workflow:
- executor
executor:
- name: Assistant
type: ReAct
persona: >-
You are expert researcher and great communicator
of complex topics using simple terms. You always
give comprehensive and extensive responses.
tools:
- Calculator
- CurrentTime
- NoAction
- MCP|Github|search_repositories
llm: ollama|qwen3:4b
temperature: 0
frequencyPenalty: 0
presencePenalty: 0
topP: 1
maxTokens: 2000
stopWords: <PAUSE>, STOP
In this configuration we are using Qwen3 4B parameter model and run it locally using Ollama. Qwen3 is a reasoning model with internal monolog. ReAct prompt imposes the structured response with <thought>
and <action>
which will be displayed in the responses in addition to reasoning section marked with <think>
.
In the example above we have added a few tools to the assistant. The Calculator
and CurrentTime
are built-in tools that are available in Elemental. The NoAction
tool is a dummy tool that does not do anything but lets agent continue the iterative sequence. The last tool is a custom tool provided with MCP server that uses the GitHub API to search for repositories. This tool requires definition of GitHub MCP server in the mcpServers
variable of .env
file. The tool name follows Elemental MCP tool naming convention MCP|Github|search_repositories
syntax.
This example can be run with the following command
python -m elemental_agents.main.elemental --config examples/09-react-agent-yaml/config-react-example.yaml --debug
We have used --debug
flag to display additional log messages that will include raw messages created by the agent. An example execution of this configuration is shown in the following video for instruction: "list github repositories for quantum computing".
Qwen3 follows well the requested structure and is able to pick the right tool and provide correct syntax parsing. With reasoning model the <thought>
section may seem redundant to the information already present in the internal reasoning monolog, however, it gives a structure for each iteration. Where smaller language models may show a deviation from the structured response is the last iteration and using the correct termination sequence ( like <result>
) to mark its final response. To mitigate the repetition of iterations we will enable relaxed ReAct mode in .env
with relaxed_react=True
.
Simply by changing the type
of the agent to type: PlanReAct
we switch from ReAct to PlanReAct prompting strategy that adds internal planning in addition to the reasoning of the agent. The agent will not only reflect on what to do next but also give it a structured plan with order of operations explicitly stated. The PlanReAct version of this example may be run with this command:
python -m elemental_agents.main.elemental --config examples/10-PlanReAct-agent-yaml/config-planreact-example.yaml --debug --save --file conversation.json
In this YAML example ( available in the examples on GitHub ) you may notice MCP|Github|*
which brings all tools from the MCP server. We have also used --save --file conversation.json
in the command. This will save the conversation with the agent to file conversation.json
at the end, when user types exit
.
Planning, Execution, Composition flow
In the previous examples we have shown how to create single agent using YAML configuration files and run them using Elemental command line interface. Now, we will look into creating agents that will be orchestrated and work through several distinct steps. By orchestration we mean managing the flow of information and invoking different member agents at the right time. The orchestration component is responsible for creating the task queue, executing the tasks, and providing the final result.
The default orchestration in Elemental is done using DynamicAgentOrchestrator
class. The orchestration is done in a sequence of steps that are executed in a specific order that are shown in the figure below.
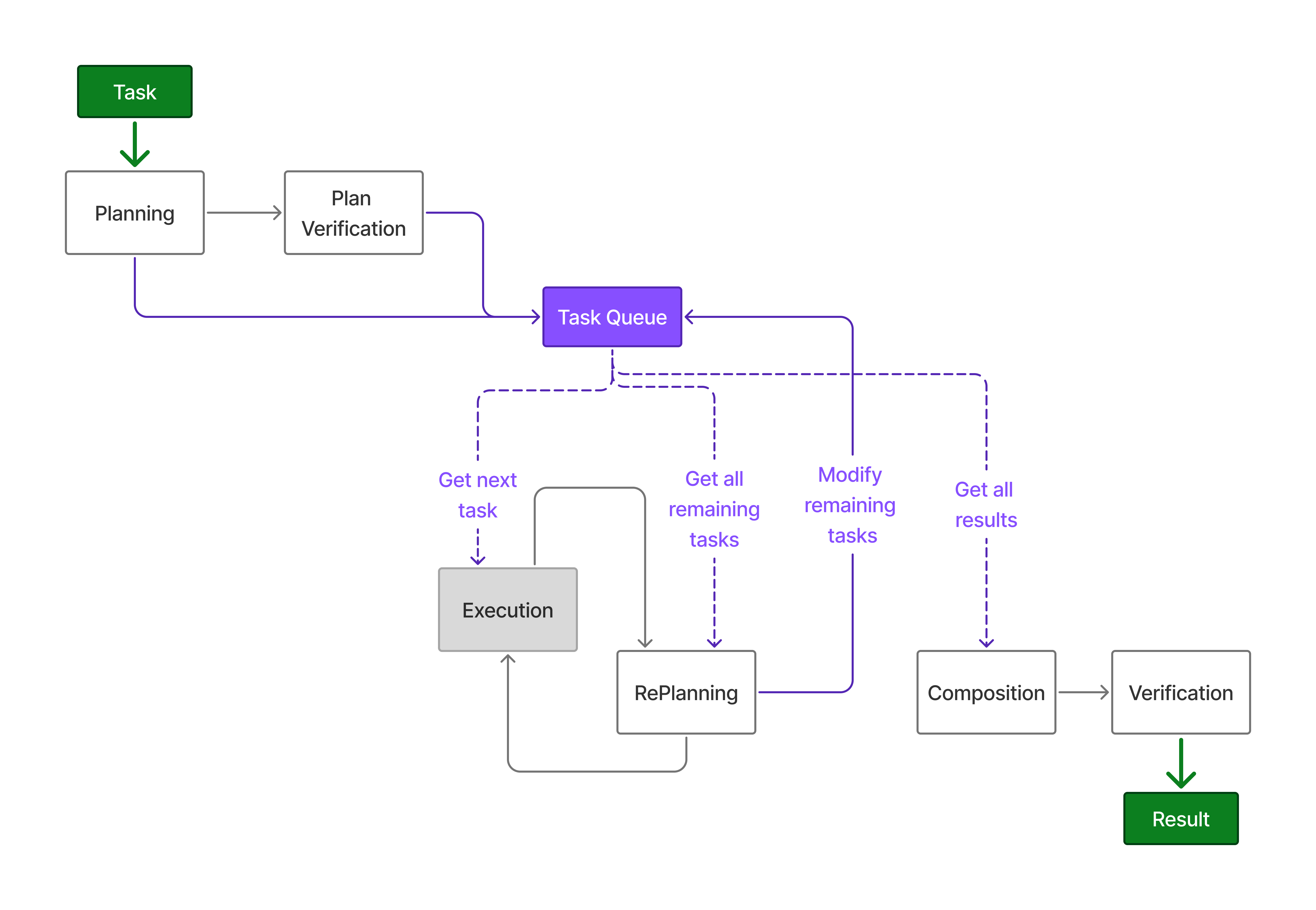
In the figure above the information flows from left to right. The white blocks represent the steps in the orchestration process. The gray block is the Execution step which is mandatory and must be present in the orchestration. In all previous examples we have used YAML configurations that included execution step and de facto we have used the orchestration process with only a single step.
In the example below we will show how to create an orchestrated team of agents that will work together to solve a problem. The orchestrated team will consist of a planner agent, executor agent, and a composition agent. The planner agent will create a plan based on the user input and the executor agent will execute the plan. The composer will be responsible for creating the final response.
workflowName: Composer Team
workflow:
- planner
- executor
- composer
planner:
- name: alice
type: Planner
persona: ""
tools: []
llm: openai|gpt-4o
temperature: 0
frequencyPenalty: 0
presencePenalty: 0
topP: 1
maxTokens: 10000
stopWords: <PAUSE>, STOP
executor:
- name: bob
type: ReAct
persona: >-
Research, developer and teacher agent. Always
very detailed in responses and due diligent in
completing tasks.
tools:
- Calculator
- CurrentTime
- NoAction
llm: openai|gpt-4o
temperature: 0
frequencyPenalty: 0
presencePenalty: 0
topP: 1
maxTokens: 10000
stopWords: <PAUSE>, STOP
composer:
- name: charlie
type: Composer
persona: >-
Research, developer and teacher agent. Always
very detailed in responses and due diligent in
completing tasks. Use markdown in your response
for more readable format. Use all information
provided and create verbose and high quality
document.
tools: []
llm: openai|gpt-4o
temperature: 0
frequencyPenalty: 0
presencePenalty: 0
topP: 1
maxTokens: 10000
stopWords: <PAUSE>, STOP
In this example we have created a team of three agents. The alice
agent is a planner agent that will create a plan based on the user input. The bob
agent is an executor agent that will execute the plan. The charlie
agent is a composer agent that will create the final response.
To illustrate using different language model we have used openai|gpt-4o
model. To use OpenAI provided models the .env
will require additional parameters.
In the .env
file you will need to add the following lines:
openai_api_key="sk-..."
openai_streaming=True
The above example may be run with the following command
python -m elemental_agents.main.elemental --config examples/11-orchestrated-team-yaml/config-orchestrated-team.yaml
The result of the command above is available in the recording below. The instruction used in the recording is: create comprehensive report on the US supreme court.
Orchestrated mode allows for more complex agent workflows. Stay tuned for more examples.